1. Introduction: Extending the RS274NGC Interpreter by Remapping Codes
1.1. A Definition: Remapping Codes
By remapping codes we mean one of the following:
-
Define the semantics of new - that is, currently unallocated - M- or G-codes
-
Redefine the semantics of a - currently limited - set of existing codes.
1.2. Why would you want to extend the RS274NGC Interpreter?
The set of codes (M,G,T,S,F) currently understood by the RS274NGC interpreter is fixed and cannot be extended by configuration options.
In particular, some of these codes implement a fixed sequence of steps to be executed. While some of these, like M6, can be moderately configured by activating or skipping some of these steps through INI file options, overall the behavior is fairly rigid. So - if your are happy with this situation, then this manual section is not for you.
In many cases, this means that supporting a non out of the box configuration or machine is either cumbersome or impossible, or requires resorting to changes at the C/C+\+ language level. The latter is unpopular for good reasons - changing internals requires in-depth understanding of interpreter internals, and moreover brings its own set of support issues. While it is conceivable that certain patches might make their way into the main LinuxCNC distribution, the result of this approach is a hodge-podge of special-case solutions.
A good example for this deficiency is tool change support in LinuxCNC: While random tool changers are well supported, it is next to impossible to reasonably define a configuration for a manual-tool change machine with, for example, an automatic tool length offset switch being visited after a tool change, and offsets set accordingly. Also, while a patch for a very specific rack tool changer exists, it has not found its way back into the main code base.
However, many of these things may be fixed by using an O-word procedure instead of a built in code - whenever the - insufficient - built in code is to be executed, call the O-word procedure instead. While possible, it is cumbersome - it requires source-editing of NGC programs, replacing all calls to the deficient code by a an O-word procedure call.
In its simplest form, a remapped code isn’t much more than a spontaneous call to an O-word procedure. This happens behind the scenes - the procedure is visible at the configuration level, but not at the NGC program level.
Generally, the behavior of a remapped code may be defined in the following ways:
-
You define a O-word subroutine which implements the desired behavior
-
Alternatively, you may employ a Python function which extends the interpreter’s behavior.
M- and G-codes, and O-words subroutine calls have some fairly different syntax.
O-word procedures, for example, take positional parameters with a specific syntax like so:
whereas M- or G-codes typically take required or optional word parameters. For instance, G76 (threading) requires the P,Z,I,J and K words, and optionally takes the R,Q,H, E and L words.
So it isn’t simply enough to say whenever you encounter code X, please call procedure Y - at least some checking and conversion of parameters needs to happen. This calls for some glue code between the new code, and its corresponding NGC procedure to execute before passing control to the NGC procedure.
This glue code is impossible to write as an O-word procedure itself, since the RS274NGC language lacks the introspective capabilities and access into interpreter internal data structures to achieve the required effect. Doing the glue code in - again - C/C+\+ would be an inflexible and therefore unsatisfactory solution.
To make a simple situation easy and a complex situation solvable, the glue issue is addressed as follows:
-
For simple situations, a built-in glue procedure (
argspec
) covers most common parameter passing requirements. -
For remapping T,M6,M61,S,F there is some standard Python glue which should cover most situations, see Standard Glue.
-
For more complex situations, one can write your own Python glue to implement new behavior.
Embedded Python functions in the Interpreter started out as glue code, but turned out very useful well beyond that. Users familiar with Python will likely find it easier to write remapped codes, glue, O-word procedures, etc. in pure Python, without resorting to the somewhat cumbersome RS274NGC language at all.
Many people are familiar with extending the Python interpreter by C/C++ modules, and this is heavily used in LinuxCNC to access Task, HAL and Interpreter internals from Python scripts. Extending Python basically means: Your Python script executes as it is in the driver seat, and may access non-Python code by importing and using extension modules written in C/C+\+. Examples for this are the LinuxCNC hal
, gcode
and emc
modules.
Embedded Python is a bit different and less commonly known: The main program is written in C/C++ and may use Python like a subroutine. This is powerful extension mechanism and the basis for the scripting extensions found in many successful software packages. Embedded Python code may access C/C+\+ variables and functions through a similar extension module method.
2. Getting started
Defining a code involves the following steps:
-
Pick a code - either use an unallocated code, or redefine an existing code.
-
Decide how parameters are handled.
-
Decide if and how results are handled.
-
Decide about the execution sequence.
2.1. Builtin Remaps
Please note that currently only some existing codes can be redefined, while there are many free codes that may be available for remapping. When developing redefined existing code, it is a good idea to start with an unassigned G- or M- code, so that you can use both an existing behavior as well as a new one. When you’re done, redefine the existing code to use your remapping configuration.
-
The current set of unused M-codes, available for user definition, can be found in the unallocated M-codes section.
-
For G-codes, see the unallocated G-codes list.
-
Existing codes that can be reassigned are listed in the remappable codes section.
There are currently two complete Python-only remaps that are available in stdglue.py:
-
ignore_m6
-
index_lathe_tool_with_wear
These are meant for use with lathe. Lathes don’t use M6 to index the tools, they use the T command.
This remap also adds wear offsets to the tool offset, e.g. T201 would index to tool 2 (with tool 2’s tool offset) and adds wear offset 1. In the tool table, tools numbers above 10000 are wear offsets, e.g. in the tool table, tool 10001 would be wear offset 1.
Here is what you need in the INI to use them:
[RS274NGC] REMAP=T python=index_lathe_tool_with_wear REMAP=M6 python=ignore_m6 [PYTHON] # where to find the Python code: # code specific for this configuration PATH_PREPEND=./ # generic support code - make sure this actually points to Python-stdglue PATH_APPEND=../../nc_files/remap_lib/python-stdglue/ # import the following Python module TOPLEVEL=toplevel.py # the higher the more verbose tracing of the Python plugin LOG_LEVEL = 0
You must also add the required Python file in your configuration folder.
2.2. Picking a code
Note that currently only a few existing codes may be redefined, whereas there are many free codes which might be made available by remapping. When developing a redefined existing code, it might be a good idea to start with an unallocated G- or M-code, so both the existing and new behavior can be exercised. When done, redefine the existing code to use your remapping setup.
2.3. Parameter handling
Let’s assume the new code will be defined by an NGC procedure, and needs some parameters, some of which might be required, others might be optional. We have the following options to feed values to the procedure:
-
Extracting words from the current block and pass them to the procedure as parameters (like
X22.34
orP47
), -
referring to INI file variables,
-
referring to global variables (like
#2200 = 47.11
or#<_global_param> = 315.2
).
The first method is preferred for parameters of dynamic nature, like positions. You need to define which words on the current block have any meaning for your new code, and specify how that is passed to the NGC procedure. Any easy way is to use the argspec statement. A custom prolog might provide better error messages.
Using to INI file variables is most useful for referring to setup information for your machine, for instance a fixed position like a tool-length sensor position. The advantage of this method is that the parameters are fixed for your configuration, regardless which NGC file you’re currently executing.
Referring to global variables is always possible, but they are easily overlooked.
Note there’s a limited supply of words which may be used as parameters, so one might need to fall back to the second and third methods if many parameters are needed.
2.4. Handling results
Your new code might succeed or fail, for instance if passed an invalid parameter combination. Or you might choose to just execute the procedure and disregard results, in which case there isn’t much work to do.
Epilog handlers help in processing results of remap procedures - see the reference section.
2.5. Execution sequencing
Executable G-code words are classified into modal groups, which also defines their relative execution behavior.
If a G-code block contains several executable words on a line, these words are executed in a predefined order of execution, not in the order they appear in block.
When you define a new executable code, the interpreter does not yet know where your code fits into this scheme. For this reason, you need to choose an appropriate modal group for your code to execute in.
2.6. An minimal example remapped code
To give you an idea how the pieces fit together, let’s explore a fairly minimal but complete remapped code definition. We choose an unallocated M-code and add the following option to the INI file:
[RS274NGC] REMAP=M400 modalgroup=10 argspec=Pq ngc=myprocedure
In a nutshell, this means:
-
The
M400
code takes a required parameterP
and an optional parameterQ
. Other words in the current block are ignored with respect to theM400
code. If theP
word is not present, fail execution with an error. -
When an
M400
code is encountered, executemyprocedure.ngc
along the other modal group 10 M-codes as per order of execution. -
The value of
P
, andQ
are available in the procedure as local named parameters. The may be referred to as#<P>
and#<Q>
. The procedure may test whether theQ
word was present with theEXISTS
built in function.
The file myprocedure.ngc
is expected to exists in the [DISPLAY]NC_FILES
or [RS274NGC]SUBROUTINE_PATH
directory.
A detailed discussion of REMAP parameters is found in the reference section below.
3. Configuring Remapping
3.1. The REMAP statement
To remap a code, define it using the REMAP
option in RS274NG
section of your INI file. Use one REMAP
line per remapped code.
The syntax of the REMAP
is:
It is an error to omit the <code> parameter.
The options of the REMAP statement are separated by whitespace. The options are keyword-value pairs and currently are:
-
modalgroup=
<modal group> -
- G-codes
-
the only currently supported modal group is 1, which is also the default value if no group is given. Group 1 means execute alongside other G-codes.
- M-codes
-
Currently supported modal groups are: 5,6,7,8,9,10. If no modalgroup is give, it defaults to 10 (execute after all other words in the block).
- T,S,F
-
for these the modal group is fixed and any
modalgroup=
option is ignored.
-
argspec=
<argspec> -
See description of the argspec parameter options. Optional.
-
ngc=
<ngc_basename> -
Basename of an O-word subroutine file name. Do not specify an .ngc extension. Searched for in the directories specified in the directory specified in
[DISPLAY]PROGRAM_PREFIX
, then in[RS274NGC]SUBROUTINE_PATH
. Mutually exclusive withpython=
. It is an error to omit bothngc=
andpython=
. -
python=
<Python function name> -
Instead of calling an ngc O-word procedure call a Python function. The function is expected to be defined in the
module_basename.oword
module. Mutually exclusive withngc=
. -
prolog=
<Python function name> -
Before executing an ngc procedure, call this Python function. The function is expected to be defined in the
module_basename.remap
module. Optional. -
epilog=
<Python function name> -
After executing an ngc procedure, call this Python function. The function is expected to be defined in the
module_basename.remap
module. Optional.
The python
, prolog
and epilog
options require the Python Interpreter plugin to be configured, and appropriate Python functions to be defined there so they can be referred to with these options.
The syntax for defining a new code, and redefining an existing code is identical.
3.2. Useful REMAP option combinations
Note that while many combinations of argspec options are possible, not all of them make sense. The following combinations are useful idioms:
-
argspec=
<words>ngc=
<procname>modalgroup=
_<group> -
The recommended way to call an NGC procedure with a standard argspec parameter conversion. Used if argspec is good enough. Note, it is not good enough for remapping the
Tx
andM6
/M61
tool change codes. -
prolog=
<pythonprolog>ngc=
<procname>epilog=
<pythonepilog>modalgroup=
<group> -
Call a Python prolog function to take any preliminary steps, then call the NGC procedure. When done, call the Python epilog function to do any cleanup or result extraction work which cannot be handled in G-code. The most flexible way of remapping a code to an NGC procedure, since almost all of the Interpreter internal variables, and some internal functions may be accessed from the prolog and epilog handlers. Also, a longer rope to hang yourselves.
-
python=
<pythonfunction>modalgroup=
<group> -
Directly call to a Python function without any argument conversion. The most powerful way of remapping a code and going straight to Python. Use this if you do not need an NGC procedure, or NGC is just getting in your way.
-
argspec=
<words>python=
<pythonfunction>modalgroup=
<group> -
Convert the argspec words and pass them to a Python function as keyword argument dictionary. Use it when you’re too lazy to investigate words passed on the block yourself.
Note that if all you want to achieve is to call some Python code from G-code, there is the somewhat easier way of calling Python functions like O-word procedures.
3.3. The argspec parameter
The argument specification (keyword argspec
) describes required and optional words to be passed to an ngc procedure, as well as optional preconditions for that code to execute.
An argspec consists of 0 or more characters of the class [@A-KMNP-Za-kmnp-z^>]
. It can by empty (like argspec=
).
An empty argspec, or no argspec argument at all implies the remapped code does not receive any parameters from the block. It will ignore any extra parameters present.
Note that RS274NGC rules still apply - for instance you may use axis words (e.g., X
, Y
, Z
) only in the context of a G-code.
Axis words may also only be used if the axis is enabled. If only XYZ are enabled, ABCUVW will not be available to be used in argspec.
Words F
, S
and T
(short FST
) will have the normal functions but will be available as variables in the remapped function. F
will set feedrate, S
will set spindle RPM, T
will trigger the tool prepare function. Words FST
should not be used if this behavior is not desired.
Words DEIJKPQR
have no predefined function and are recommended for use as argspec parameters.
-
ABCDEFHIJKPQRSTUVWXYZ
-
Defines a required word parameter: an uppercase letter specifies that the corresponding word must be present in the current block. The word`s value will be passed as a local named parameter with a corresponding name. If the
@
character is present in the argspec, it will be passed as positional parameter, see below. -
abcdefhijkpqrstuvwxyz
-
Defines an optional word parameter: a lowercase letter specifies that the corresponding word may be present in the current block. If the word is present, the word’s value will be passed as a local named parameter. If the
@
character is present in the argspec, it will be passed as positional parameter, see below. -
@
-
The
@
(at-sign) tells argspec to pass words as positional parameters, in the order defined following the@
option. Note that when using positional parameter passing, a procedure cannot tell whether a word was present or not, see example below.
this helps with packaging existing NGC procedures as remapped codes. Existing procedures do expect positional parameters. With the @ option, you can avoid rewriting them to refer to local named parameters. |
-
^
-
The
^
(caret) character specifies that the current spindle speed must be greater than zero (spindle running), otherwise the code fails with an appropriate error message. -
>
-
The
>
(greater-than) character specifies that the current feed must be greater than zero, otherwise the code fails with an appropriate error message. -
n
-
The
n
(greater-than) character specifies to pass the current line number in the `n`local named parameter.
By default, parameters are passed as local named parameter to an NGC procedure. These local parameters appear as already set when the procedure starts executing, which is different from existing semantics (local variables start out with value 0.0 and need to be explicitly assigned a value).
Optional word parameters may be tested for presence by the EXISTS(#<word>)
idiom.
Assume the code is defined as
REMAP=M400 modalgroup=10 argspec=Pq ngc=m400
and m400.ngc
looks as follows:
-
Executing
M400
will fail with the messageuser-defined M400: missing: P
. -
Executing
M400 P123
will displayP word=123.000000
. -
Executing
M400 P123 Q456
will displayP word=123.000000
andQ word set: 456.000000
.
Assume the code is defined as
REMAP=M410 modalgroup=10 argspec=@PQr ngc=m410
and m410.ngc
looks as follows:
-
Executing
M410 P10
will displaym410.ngc: [1]=10.000000 [2]=0.000000
. -
Executing
M410 P10 Q20
will displaym410.ngc: [1]=10.000000 [2]=20.000000
.
you lose the capability to distinguish more than one optional parameter word, and you cannot tell whether an optional parameter was present but had the value 0, or was not present at all. |
It’s possible to define new codes without any NGC procedure. Here’s a simple first example, a more complex one can be found in the next section.
Assume the code is defined as
REMAP=G88.6 modalgroup=1 argspec=XYZp python=g886
This instructs the interpreter to execute the Python function g886
in the module_basename.remap
module, which might look like so:
from interpreter import INTERP_OK from emccanon import MESSAGE def g886(self, **words): for key in words: MESSAGE("word '%s' = %f" % (key, words[key])) if words.has_key('p'): MESSAGE("the P word was present") MESSAGE("comment on this line: '%s'" % (self.blocks[self.remap_level].comment)) return INTERP_OK
Try this with out with: g88.6 x1 y2 z3 g88.6 x1 y2 z3 p33 (a comment here)
You’ll notice the gradual introduction of the embedded Python environment - see here for details. Note that with Python remapping functions, it make no sense to have Python prolog or epilog functions since it is executing a Python function in the first place.
The interpreter
and emccanon
modules expose most of the Interpreter and some Canon internals, so many things which so far required coding in C/C+\+ can be now be done in Python.
The following example is based on the nc_files/involute.py
script - but canned as a G-code with some parameter extraction and checking. It also demonstrates calling the interpreter recursively (see self.execute()
).
Assuming a definition like so (NB: this does not use argspec):
REMAP=G88.1 modalgroup=1 py=involute
The involute
function in python/remap.py
listed below does all word extraction from the current block directly. Note that interpreter errors can be translated to Python exceptions. Remember this is readahead time - execution time errors cannot be trapped this way.
import sys import traceback from math import sin,cos from interpreter import * from emccanon import MESSAGE from util import lineno, call_pydevd # raises InterpreterException if execute() or read() fails throw_exceptions = 1 def involute(self, **words): """ remap function with raw access to Interpreter internals """ if self.debugmask & 0x20000000: call_pydevd() # USER2 debug flag if equal(self.feed_rate,0.0): return "feedrate > 0 required" if equal(self.speed[0], 0.0): return "spindle speed > 0 required" plunge = 0.1 # if Z word was given, plunge - with reduced feed # inspect controlling block for relevant words c = self.blocks[self.remap_level] x0 = c.x_number if c.x_flag else 0 y0 = c.y_number if c.y_flag else 0 a = c.p_number if c.p_flag else 10 old_z = self.current_z if self.debugmask & 0x10000000: print("x0=%f y0=%f a=%f old_z=%f" % (x0,y0,a,old_z)) try: #self.execute("G3456") # would raise InterpreterException self.execute("G21",lineno()) self.execute("G64 P0.001",lineno()) self.execute("G0 X%f Y%f" % (x0,y0),lineno()) if c.z_flag: feed = self.feed_rate self.execute("F%f G1 Z%f" % (feed * plunge, c.z_number),lineno()) self.execute("F%f" % (feed),lineno()) for i in range(100): t = i/10. x = x0 + a * (cos(t) + t * sin(t)) y = y0 + a * (sin(t) - t * cos(t)) self.execute("G1 X%f Y%f" % (x,y),lineno()) if c.z_flag: # retract to starting height self.execute("G0 Z%f" % (old_z),lineno()) except InterpreterException,e: msg = "%d: '%s' - %s" % (e.line_number,e.line_text, e.error_message) return msg return INTERP_OK
The examples described so far can be found in configs/sim/axis/remap/getting-started with complete working configurations.
4. Upgrading an existing configuration for remapping
The minimal prerequisites for using REMAP
statements are as follows:
-
The Python plug in must be activated by specifying a
[PYTHON]TOPLEVEL=<path-to-toplevel-script>
in the INI file. -
The toplevel script needs to import the
remap
module, which can be initially empty, but the import needs to be in place. -
The Python interpreter needs to find the remap.py module above, so the path to the directory where your Python modules live needs to be added with
[PYTHON]PATH_APPEND=<path-to-your-local-Python-directory>
-
Recommended: import the
stdglue
handlers in theremap
module. In this case Python also needs to findstdglue.py
- we just copy it from the distribution so you can make local changes as needed. Depending on your installation the path tostdglue.py
might vary.
Assuming your configuration lives under /home/user/xxx
and the INI file is /home/user/xxx/xxx.ini
, execute the following commands.
$ cd /home/user/xxx $ mkdir python $ cd python $ cp /usr/share/linuxcnc/ncfiles/remap_lib/python-stdglue/stdglue.py . $ echo 'from stdglue import *' >remap.py $ echo 'import remap' >toplevel.py
Now edit ``/home/user/``xxx``/``xxx``.ini`` and add the following:
[PYTHON] TOPLEVEL=/home/user/xxx/python/toplevel.py PATH_APPEND=/home/user/xxx/python
Now verify that LinuxCNC comes up with no error messages - from a terminal window execute:
$ cd /home/user/xxx
$ linuxcnc xxx.ini
5. Remapping tool change-related codes: T, M6, M61
5.1. Overview
If you are unfamiliar with LinuxCNC internals, first read the How tool change currently works section (dire but necessary).
Note than when remapping an existing code, we completely disable this codes' built-in functionality of the interpreter.
So our remapped code will need to do a bit more than just generating some commands to move the machine as we like - it will also need to replicate those steps from this sequence which are needed to keep the interpreter and task
happy.
However, this does not affect the processing of tool change-related commands in task
and iocontrol
. This means when we execute step 6b this will still cause iocontrol to do its thing.
Decisions, decisions:
-
Do we want to use an O-word procedure or do it all in Python code?
-
Is the
iocontrol
HAL sequence (tool-prepare/tool-prepared and tool-change/tool-changed pins) good enough or do we need a different kind of HAL interaction for our tool changer (for example: more HAL pins involved with a different interaction sequence)?
Depending on the answer, we have four different scenarios:
-
When using an O-word procedure, we need prolog and epilog functions.
-
If using all Python code and no O-word procedure, a Python function is enough.
-
When using the
iocontrol
pins, our O-word procedure or Python code will contain mostly moves. -
When we need a more complex interaction than offered by
iocontrol
, we need to completely define our own interaction, usingmotion.digital*
andmotion.analog*
pins, and essentially ignore theiocontrol
pins by looping them.
If you hate O-word procedures and love Python, you are free to do it all in Python, in which case you would just have a python= <function> spec in the REMAP statement. But assuming most folks would be interested in using O-word procedures because they are more familiar with that, we’ll do that as the first example. |
So the overall approach for our first example will be:
-
We’d like to do as much as possible with G-code in an O-word procedure for flexibility. That includes all HAL interaction which would normally be handled by
iocontrol
- because we rather would want to do clever things with moves, probes, HAL pin I/O and so forth. -
We’ll try to minimize Python code to the extent needed to keep the interpreter happy, and cause
task
to actually do anything. That will go into theprolog
andepilog
Python functions.
5.2. Understanding the role of iocontrol
with remapped tool change codes
iocontrol
provides two HAL interaction sequences we might or might not use:
-
When the NML message queued by a SELECT_TOOL() canon command is executed, this triggers the "raise tool-prepare and wait for tool-prepared to become high" HAL sequence in
iocontrol
, besides setting the XXXX pins -
When the NML message queued by the CHANGE_TOOL() canon command is executed, this triggers the "raise tool-change and wait for tool-changed to become high" HAL sequence in
iocontrol
, besides setting the XXXX pins
What you need to decide is whether the existing iocontrol
HAL sequences are sufficient to drive your changer. Maybe you need a different interaction sequence - for instance more HAL pins, or maybe a more complex interaction. Depending on the answer, we might continue to use the existing iocontrol
HAL sequences, or define our own ones.
For the sake of documentation, we’ll disable these iocontrol
sequences, and roll our own - the result will look and feel like the existing interaction, but now we have complete control over them because they are executed in our own O-word procedure.
So what we’ll do is use some motion.digital-*
and motion.analog-*
pins, and the associated M62
.. M68
commands to do our own HAL interaction in our O-word procedure, and those will effectively replace the iocontrol
tool-prepare/tool-prepared and tool-change/tool-changed sequences. So we’ll define our pins replacing existing iocontrol
pins functionally, and go ahead and make the iocontrol
interactions a loop. We’ll use the following correspondence in our example:
iocontrol
pin correspondence in the examples
iocontrol.0 pin |
motion pin |
---|---|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Let us assume you want to redefine the M6 command, and replace it by an O-word procedure, but other than that things should continue to work.
So what our O-word procedure would do is to replace the steps outlined here. Looking through these steps you’ll find that NGC code can be used for most of them, but not all. So the stuff NGC can’t handle will be done in Python prolog and epilog functions.
5.3. Specifying the M6 replacement
To convey the idea, we just replace the built in M6 semantics with our own. Once that works, you may go ahead and place any actions you see fit into the O-word procedure.
Going through the steps, we find:
-
check for T command already executed - execute in Python prolog
-
check for cutter compensation being active - execute in Python prolog
-
stop the spindle if needed - can be done in NGC
-
quill up - can be done in NGC
-
if TOOL_CHANGE_AT_G30 was set:
-
move the A, B and C indexers if applicable - can be done in NGC
-
generate rapid move to the G30 position - can be done in NGC
-
-
send a CHANGE_TOOL Canon command to
task
- execute in Python epilog -
set the numberer parameters 5400-5413 according to the new tool - execute in Python epilog
-
signal to
task
to stop calling the interpreter for readahead until tool change complete - execute in Python epilog
So we need a prolog, and an epilog. Lets assume our INI file incantation of the M6 remap looks as follows:
REMAP=M6 modalgroup=6 prolog=change_prolog ngc=change epilog=change_epilog
So the prolog covering steps 1 and 2 would look like so - we decide to pass a few variables to the remap procedure which can be inspected and changed there, or used in a message. Those are: tool_in_spindle
, selected_tool
(tool numbers) and their respective tooldata indices current_pocket
and selected_pocket
:
The legacy names selected_pocket and current_pocket actually reference a sequential tooldata index for tool items loaded from a tool table ([EMCIO]TOOL_TABLE) or via a tooldata database ([EMCIO]DB_PROGRAM). |
def change_prolog(self, **words): try: if self.selected_pocket < 0: return "M6: no tool prepared" if self.cutter_comp_side: return "Cannot change tools with cutter radius compensation on" self.params["tool_in_spindle"] = self.current_tool self.params["selected_tool"] = self.selected_tool self.params["current_pocket"] = self.current_pocket self.params["selected_pocket"] = self.selected_pocket return INTERP_OK except Exception as e: return "M6/change_prolog: {}".format(e)
You will find that most prolog functions look very similar:
-
First test that all preconditions for executing the code hold, then
-
prepare the environment - inject variables and/or do any preparatory processing steps which cannot easily be done in NGC code;
-
then hand off to the NGC procedure by returning INTERP_OK.
Our first iteration of the O-word procedure is unexciting - just verify we got parameters right, and signal success by returning a positive value; steps 3-5 would eventually be covered here (see here for the variables referring to INI file settings):
Assuming success of change.ngc
, we need to mop up steps 6-8:
def change_epilog(self, **words): try: if self.return_value > 0.0: # commit change self.selected_pocket = int(self.params["selected_pocket"]) emccanon.CHANGE_TOOL(self.selected_pocket) # cause a sync() self.tool_change_flag = True self.set_tool_parameters() return INTERP_OK else: return "M6 aborted (return code %.1f)" % (self.return_value) except Exception, e: return "M6/change_epilog: %s" % (e)
This replacement M6 is compatible with the built in code, except steps 3-5 need to be filled in with your NGC code.
Again, most epilogs have a common scheme:
-
First, determine whether things went right in the remap procedure,
-
then do any commit and cleanup actions which can’t be done in NGC code.
5.4. Configuring iocontrol
with a remapped M6
Note that the sequence of operations has changed: we do everything required in the O-word procedure - including any HAL pin setting/reading to get a changer going, and to acknowledge a tool change - likely with motion.digital-*
and motion-analog-*
IO pins. When we finally execute the CHANGE_TOOL()
command, all movements and HAL interactions are already completed.
Normally only now iocontrol
would do its thing as outlined here. However, we don’t need the HAL pin wiggling anymore - all iocontrol
is left to do is to accept we’re done with prepare and change.
This means that the corresponding iocontrol
pins have no function any more. Therefore, we configure iocontrol
to immediately acknowledge a change by configuring like so:
If you for some reason want to remap Tx
(prepare), the corresponding iocontrol
pins need to be looped as well.
5.5. Writing the change and prepare O-word procedures
The standard prologs and epilogs found in ncfiles/remap_lib/python-stdglue/stdglue.py
pass a few exposed parameters to the remap procedure.
An exposed parameter is a named local variable visible in a remap procedure which corresponds to interpreter-internal variable, which is relevant for the current remap. Exposed parameters are set up in the respective prolog, and inspected in the epilog. They can be changed in the remap procedure and the change will be picked up in the epilog. The exposed parameters for remappable built in codes are:
-
T
(prepare_prolog):#<tool>
,#<pocket>
-
M6
(change_prolog):#<tool_in_spindle>
,#<selected_tool>
,#<current_pocket>
,#<selected_pocket>
-
M61
(settool_prolog):#<tool>
,#<pocket>
-
S
(setspeed_prolog):#<speed>
-
F
(setfeed_prolog):#<feed>
If you have specific needs for extra parameters to be made visible, that can simply be added to the prolog - practically all of the interpreter internals are visible to Python.
5.6. Making minimal changes to the built in codes, including M6
Remember that normally remapping a code completely disables all internal processing for that code.
However, in some situations it might be sufficient to add a few codes around the existing M6
built in implementation, like a tool length probe, but other than that retain the behavior of the built in M6
.
Since this might be a common scenario, the built in behavior of remapped codes has been made available within the remap procedure. The interpreter detects that you are referring to a remapped code within the procedure which is supposed to redefine its behavior. In this case, the built in behavior is used - this currently is enabled for the set: M6
, M61
,T
, S
, F
. Note that otherwise referring to a code within its own remap procedure would be a error - a remapping recursion
.
Slightly twisting a built in would look like so (in the case of M6
):
REMAP=M6 modalgroup=6 ngc=mychange
When redefining a built-in code, do not specify any leading zeroes in G- or M-codes - for example, say REMAP=M1 .. , not REMAP=M01 ... . |
See the configs/sim/axis/remap/extend-builtins
directory for a complete configuration, which is the recommended starting point for own work when extending built in codes.
5.7. Specifying the T (prepare) replacement
If you’re confident with the default implementation, you wouldn’t need to do this. But remapping is also a way to work around deficiencies in the current implementation, for instance to not block until the "tool-prepared" pin is set.
What you could do, for instance, is:
- In a remapped T, just set the equivalent of the tool-prepare
pin, but not wait for tool-prepared
here.
- In the corresponding remapped M6, wait for the tool-prepared
at the very beginning of the O-word procedure.
Again, the iocontrol
tool-prepare/tool-prepared pins would be unused and replaced by motion.*
pins, so those would pins must be looped:
So, here’s the setup for a remapped T:
REMAP=T prolog=prepare_prolog epilog=prepare_epilog ngc=prepare
def prepare_prolog(self,**words): try: cblock = self.blocks[self.remap_level] if not cblock.t_flag: return "T requires a tool number" tool = cblock.t_number if tool: (status, pocket) = self.find_tool_pocket(tool) if status != INTERP_OK: return "T%d: pocket not found" % (tool) else: pocket = -1 # this is a T0 - tool unload # these variables will be visible in the ngc O-word sub # as #<tool> and #<pocket> local variables, and can be # modified there - the epilog will retrieve the changed # values self.params["tool"] = tool self.params["pocket"] = pocket return INTERP_OK except Exception, e: return "T%d/prepare_prolog: %s" % (int(words['t']), e)
The minimal ngc prepare procedure again looks like so:
And the epilog:
def prepare_epilog(self, **words): try: if self.return_value > 0: self.selected_tool = int(self.params["tool"]) self.selected_pocket = int(self.params["pocket"]) emccanon.SELECT_TOOL(self.selected_tool) return INTERP_OK else: return "T%d: aborted (return code %.1f)" % (int(self.params["tool"]),self.return_value) except Exception, e: return "T%d/prepare_epilog: %s" % (tool,e)
The functions prepare_prolog and prepare_epilog are part of the standard glue provided by nc_files/remap_lib/python-stdglue/stdglue.py. This module is intended to cover most standard remapping situations in a common way.
5.8. Error handling: dealing with abort
The built in tool change procedure has some precautions for dealing with a program abort, e.g., by hitting escape in AXIS during a change. Your remapped function has none of this, therefore some explicit cleanup might be needed if a remapped code is aborted. In particular, a remap procedure might establish modal settings which are undesirable to have active after an abort. For instance, if your remap procedure has motion codes (G0,G1,G38..) and the remap is aborted, then the last modal code will remain active. However, you very likely want to have any modal motion canceled when the remap is aborted.
The way to do this is by using the [RS274NGC]ON_ABORT_COMMAND
feature. This INI option specifies a O-word procedure call which is executed if task
for some reason aborts program execution. on_abort
receives a single parameter indicating the cause for calling the abort procedure, which might be used for conditional cleanup.
The reasons are defined in nml_intf/emc.hh
EMC_ABORT_TASK_EXEC_ERROR = 1, EMC_ABORT_AUX_ESTOP = 2, EMC_ABORT_MOTION_OR_IO_RCS_ERROR = 3, EMC_ABORT_TASK_STATE_OFF = 4, EMC_ABORT_TASK_STATE_ESTOP_RESET = 5, EMC_ABORT_TASK_STATE_ESTOP = 6, EMC_ABORT_TASK_STATE_NOT_ON = 7, EMC_ABORT_TASK_ABORT = 8, EMC_ABORT_INTERPRETER_ERROR = 9, // interpreter failed during readahead EMC_ABORT_INTERPRETER_ERROR_MDI = 10, // interpreter failed during MDI execution EMC_ABORT_USER = 100 // user-defined abort codes start here
[RS274NGC] ON_ABORT_COMMAND=O <on_abort> call
The suggested on_abort procedure would look like so (adapt to your needs):
Never use an M2 in a O-word subroutine, including this one. It will cause hard-to-find errors. For instance, using an M2 in a subroutine will not end the subroutine properly and will leave the subroutine NGC file open, not your main program. |
Make sure on_abort.ngc
is along the interpreter search path (recommended location: SUBROUTINE_PATH
so as not to clutter your NC_FILES
directory with internal procedures).
Statements in that procedure typically would assure that post-abort any state has been cleaned up, like HAL pins properly reset. For an example, see configs/sim/axis/remap/rack-toolchange
.
Note that terminating a remapped code by returning INTERP_ERROR from the epilog (see previous section) will also cause the on_abort
procedure to be called.
5.9. Error handling: failing a remapped code NGC procedure
If you determine in your handler procedure that some error condition occurred, do not use M2
to end your handler - see above:
If displaying an operator error message and stopping the current program is good enough, use the (abort, `__<message>__
)` feature to terminate the handler with an error message. Note that you can substitute numbered, named, INI and HAL parameters in the text like in this example (see also tests/interp/abort-hot-comment/test.ngc
):
INI and HAL variable expansion is optional and can be disabled in the INI file |
If more fine grained recovery action is needed, use the idiom laid out in the previous example:
-
Define an epilog function, even if it is just to signal an error condition,
-
pass a negative value from the handler to signal the error,
-
inspect the return value in the epilog function,
-
take any recovery action needed,
-
return the error message string from the handler, which will set the interpreter error message and abort the program (pretty much like
abort, message=
).
This error message will be displayed in the UI, and returning INTERP_ERROR will cause this error handled like any other runtime error.
Note that both (abort,
<msg> )
and returning INTERP_ERROR from an epilog will cause any ON_ABORT handler to be called as well if defined (see previous section).
6. Remapping other existing codes:
6.1. Automatic gear selection be remapping S (set spindle speed)
A potential use for a remapped S code would be automatic gear selection depending on speed. In the remap procedure one would test for the desired speed attainable given the current gear setting, and change gears appropriately if not.
6.2. Adjusting the behavior of M0, M1
A use case for remapping M0/M1 would be to customize the behavior of the existing code. For instance, it could be desirable to turn off the spindle, mist and flood during an M0 or M1 program pause, and turn these settings back on when the program is resumed.
For a complete example doing just that, see configs/sim/axis/remap/extend-builtins/
, which adapts M1 as laid out above.
6.3. Adjusting the behavior of M7, M8, M9
An example for remapping the built in behavior of M7/M8/M9 is the option to pass optional arguments like a P word for more complex coolant control (eg through tool vs external coolant flow).
See configs/sim/axis/remap/extend-builtins/
, for an example of such an extension of the built in behavior for M7,M8 and M9.
7. Creating new G-code cycles
A G-code cycle as used here is meant to behave as follows:
-
On first invocation, the associated words are collected and the G-code cycle is executed.
-
If subsequent lines just continue parameter words applicable to this code, but no new G-code, the previous G-code is re-executed with the parameters changed accordingly.
An example: Assume you have G84.3
defined as remapped G-code cycle with the following INI segment (see here for a detailed description of cycle_prolog
and cycle_epilog
):
[RS274NGC] # A cycle with an O-word procedure: G84.3 <X- Y- Z- Q- P-> REMAP=G84.3 argspec=xyzabcuvwpr prolog=cycle_prolog ngc=g843 epilog=cycle_epilog modalgroup=1
Executing the following lines:
causes the following (note R is sticky, and Z is sticky since the plane is XY):
-
g843.ngc
is called with words x=1, y=2, z=3, r=1 -
g843.ngc
is called with words x=3, y=4, z=3, p=2, r=1 -
g843.ngc
is called with words x=6, y=7, z=3, r=1 -
The
G84.3
cycle is canceled.
Besides creating new cycles, this provides an easy method for repackaging existing G-codes which do not behave as cycles. For instance, the G33.1
Rigid Tapping code does not behave as a cycle. With such a wrapper, a new code can be easily created which uses G33.1
but behaves as a cycle.
See configs/sim/axis/remap/cycle for a complete example of this feature. It contains two cycles, one with an NGC procedure like above, and a cycle example using just Python.
8. Configuring Embedded Python
The Python plugin serves both the interpreter, and task
if so configured, and hence has its own section PYTHON
in the INI file.
8.1. Python plugin : INI file configuration
-
[PYTHON]
-
-
TOPLEVEL =
<filename> -
Filename of the initial Python script to execute on startup. This script is responsible for setting up the package name structure, see below.
-
PATH_PREPEND =
<directory> -
Prepend this directory to
PYTHON_PATH
. A repeating group. -
PATH_APPEND =
<directory> -
Append this directory to
PYTHON_PATH
. A repeating group. -
LOG_LEVEL =
<integer> -
Log level of plugin-related actions. Increase this if you suspect problems. Can be very verbose.
-
RELOAD_ON_CHANGE =
[0|1] -
Reload the TOPLEVEL script if the file was changed. Handy for debugging but currently incurs some runtime overhead. Turn this off for production configurations.
-
8.2. Executing Python statements from the interpreter
For ad-hoc execution of commands the Python hot comment has been added. Python output by default goes to stdout, so you need to start LinuxCNC from a terminal window to see results. Example for the MDI window:
;py,print(2*3)
Note that the interpreter instance is available here as this
, so you could also run:
;py,print(this.tool_table[0].toolno)
Here is an approach to use an O word subroutine to read a preference file entry and add it as a Gcode parameter.
9. Programming Embedded Python in the RS274NGC Interpreter
9.1. The Python plugin namespace
The namespace is expected to be laid out as follows:
-
oword
-
Any callables in this module are candidates for Python O-word procedures. Note that the Python
oword
module is checked before testing for a NGC procedure with the same name - in effect names inoword
will hide NGC files of the same basename. -
remap
-
Python callables referenced in an argspec
prolog
,epilog
orpython
option are expected to be found here. -
namedparams
-
Python functions in this module extend or redefine the namespace of predefined named parameters, see adding predefined parameters.
9.2. The Interpreter as seen from Python
The interpreter is an existing C++ class (Interp) defined in src/emc/rs274ngc. Conceptually all oword.<function>
and remap.<function>
Python calls are methods of this Interp class, although there is no explicit Python definition of this class (it is a Boost.Python wrapper instance) and hence receive the as the first parameter self
which can be used to access internals.
9.3. The Interpreter __init__
and __delete__
functions
If the TOPLEVEL
module defines a function __init__
, it will be called once the interpreter is fully configured (INI file read, and state synchronized with the world model).
If the TOPLEVEL
module defines a function __delete__
, it will be called once before the interpreter is shutdown and after the persistent parameters have been saved to the PARAMETER_FILE
.
Note_ at this time, the __delete__
handler does not work for interpreter instances created by importing the gcode
module. If you need an equivalent functionality there (which is quite unlikely), please consider the Python atexit
module.
# this would be defined in the TOPLEVEL module def __init__(self): # add any one-time initialization here if self.task: # this is the milltask instance of interp pass else: # this is a non-milltask instance of interp pass def __delete__(self): # add any cleanup/state saving actions here if self.task: # as above pass else: pass
This function may be used to initialize any Python-side attributes which might be needed later, for instance in remap or O-word functions, and save or restore state beyond what PARAMETER_FILE
provides.
If there are setup or cleanup actions which are to happen only in the milltask Interpreter instance (as opposed to the interpreter instance which sits in the gcode
Python module and serves preview/progress display purposes but nothing else), this can be tested for by evaluating self.task.
An example use of __init__
and __delete__
can be found in configs/sim/axis/remap/cycle/python/toplevel.py
initialising attributes, needed to handle cycles in ncfiles/remap_lib/python-stdglue/stdglue.py
(and imported into configs/sim/axis/remap/cycle/python/remap.py
).
9.4. Calling conventions: NGC to Python
Python code is called from NGC in the following situations:
-
during normal program execution:
-
when an O-word call like
O<proc> call
is executed and the nameoword.proc
is defined and callable -
when a comment like
;py,<Python statement>
is executed. -
during execution of a remapped code: any
prolog=
,python=
andepilog=
handlers.
-
Arguments:
-
self
-
The interpreter instance.
-
*args
-
The list of actual positional parameters. Since the number of actual parameters may vary, it is best to use this style of declaration:
# this would be defined in the oword module def mysub(self, *args): print("number of parameters passed:", len(args)) for a in args: print(a)
Just as NGC procedures may return values, so do O-word Python subroutines. They are expected to either return
-
no value (no
return
statement or the valueNone
), -
a float or int value,
-
a string, this means this is an error message, abort the program. Works like
(abort, msg)
.
Any other return value type will raise a Python exception.
In a calling NGC environment, the following predefined named parameters are available:
-
#<value>
-
Value returned by the last procedure called. Initialized to 0.0 on startup. Exposed in Interp as
self.return_value
(float). -
#<value_returned>
-
Indicates the last procedure called did
return
orendsub
with an explicit value. 1.0 if true. Set to 0.0 on eachcall
. Exposed in Interp wasself.value_returned
(int).
See also tests/interp/value-returned
for an example.
Arguments are:
-
self
-
The interpreter instance.
-
words
-
Keyword parameter dictionary. If an argspec was present, words are collected from the current block accordingly and passed in the dictionary for convenience (the words could as well be retrieved directly from the calling block, but this requires more knowledge of interpreter internals). If no argspec was passed, or only optional values were specified and none of these was present in the calling block, this dict is empty. Word names are converted to lowercase.
Example call:
def minimal_prolog(self, **words): # in remap module print(len(words)," words passed") for w in words: print("%s: %s" % (w, words[w])) if words['p'] < 78: # NB: could raise an exception if p were optional return "failing miserably" return INTERP_OK
Return values:
-
INTERP_OK
-
Return this on success. You need to import this from
interpreter
. - a message text
-
Returning a string from a handler means this is an error message, abort the program. Works like
(abort,
<msg>)
.
Arguments are:
-
self
-
The interpreter instance.
-
words
-
Keyword parameter dictionary. The same kwargs dictionary as prologs and epilogs (see above).
The minimum python=
function example:
def useless(self, **words): # in remap module return INTERP_OK
Return values:
-
INTERP_OK
-
Return this on success
- a message text
-
Returning a string from a handler means this is an error message, abort the program. Works like
(abort,
<msg>)
.
If the handler needs to execute a queuebuster operation (tool change, probe, HAL pin reading) then it is supposed to suspend execution with the following statement:
-
yield INTERP_EXECUTE_FINISH
-
This signals
task
to stop read ahead, execute all queued operations, execute the queue-buster operation, synchronize interpreter state with machine state, and then signal the interpreter to continue. At this point the function is resumed at the statement following theyield ..
statement.
Queue busters interrupt a procedure at the point where such an operation is called, hence the procedure needs to be restarted after the interpreter synch(). When this happens the procedure needs to know if it is restarted, and where to continue. The Python generator method is used to deal with procedure restart.
This demonstrates call continuation with a single point-of-restart:
def read_pin(self,*args): # wait 5secs for digital-input 00 to go high emccanon.WAIT(0,1,2,5.0) # cede control after executing the queue buster: yield INTERP_EXECUTE_FINISH # post-sync() execution resumes here: pin_status = emccanon.GET_EXTERNAL_DIGITAL_INPUT(0,0); print("pin status=",pin_status)
The yield feature is fragile. The following restrictions apply to the usage of yield INTERP_EXECUTE_FINISH: |
-
Python code executing a yield INTERP_EXECUTE_FINISH must be part of a remap procedure. Yield does not work in a Python oword procedure.
-
A Python remap subroutine containing yield INTERP_EXECUTE_FINISH statement may not return a value, as with normal Python yield statements.
-
Code following a yield may not recursively call the interpreter, like with
self.execute("<mdi command>")
. This is an architectural restriction of the interpreter and is not fixable without a major redesign.
9.5. Calling conventions: Python to NGC
NGC code is executed from Python when
-
the method
self.execute(<NGC code>[,<line number>])
is executed, or -
during execution of a remapped code, if a
prolog=
function is defined, the NGC procedure given inngc=
is executed immediately thereafter.
The prolog handler does not call the handler, but it prepares its call environment, for instance by setting up predefined local parameters.
Conceptually a prolog and an epilog execute at the same call level like the O-word procedure, that is after the subroutine call is set up, and before the subroutine endsub or return.
This means that any local variable created in a prolog will be a local variable in the O-word procedure, and any local variables created in the O-word procedure are still accessible when the epilog executes.
The self.params
array handles reading and setting numbered and named parameters. If a named parameter begins with _
(underscore), it is assumed to be a global parameter; if not, it is local to the calling procedure. Also, numbered parameters in the range 1..30 are treated like local variables; their original values are restored on return/endsub from an O-word procedure.
Here is an example remapped code demonstrating insertion and extraction of parameters into/from the O-word procedure:
REMAP=m300 prolog=insert_param ngc=testparam epilog=retrieve_param modalgroup=10
def insert_param(self, **words): # in the remap module print("insert_param call level=",self.call_level) self.params["myname"] = 123 self.params[1] = 345 self.params[2] = 678 return INTERP_OK def retrieve_param(self, **words): print("retrieve_param call level=",self.call_level) print("#1=", self.params[1]) print("#2=", self.params[2]) try: print("result=", self.params["result"]) except Exception,e: return "testparam forgot to assign #<result>" return INTERP_OK
self.params()
returns a list of all variable names currently defined. Since myname
is local, it goes away after the epilog finishes.
You can recursively call the interpreter from Python code as follows:
self.execute(<NGC code>[,<line number>])
Examples:
self.execute("G1 X%f Y%f" % (x,y)) self.execute("O <myprocedure> call", currentline)
You might want to test for the return value being < INTERP_MIN_ERROR
. If you are using lots of execute() statements, it is probably easier to trap InterpreterException as shown below.
CAUTION:
The parameter insertion/retrieval method described in the previous section does not work in this case. It is good enough for just
-
executing simple NGC commands or a procedure call and
-
advanced introspection into the procedure, and
-
passing of local named parameters is not needed.
The recursive call feature is fragile.
if interpreter.throw_exceptions
is nonzero (default 1), and self.execute() returns an error, the exception InterpreterException
is raised. InterpreterException has the following attributes:
-
line_number
-
where the error occurred
-
line_text
-
the NGC statement causing the error
-
error_message
-
the interpreter’s error message
Errors can be trapped in the following Pythonic way:
import interpreter interpreter.throw_exceptions = 1 ... try: self.execute("G3456") # raise InterpreterException except InterpreterException,e: msg = "%d: '%s' - %s" % (e.line_number,e.line_text, e.error_message) return msg # replace builtin error message
The canon layer is practically all free functions. Example:
import emccanon def example(self,*args): .... emccanon.STRAIGHT_TRAVERSE(line,x0,y0,z0,0,0,0,0,0,0) emccanon.STRAIGHT_FEED(line,x1,y1,z1,0,0,0,0,0,0) ... return INTERP_OK
The actual canon functions are declared in src/emc/nml_intf/canon.hh
and implemented in src/emc/task/emccanon.cc
. The implementation of the Python functions can be found in src/emc/rs274ncg/canonmodule.cc
.
9.6. Built in modules
The following modules are built in:
-
interpreter
-
Exposes internals of the Interp class. See
src/emc/rs274ngc/interpmodule.cc
, and thetests/remap/introspect
regression test. -
emccanon
-
Exposes most calls of
src/emc/task/emccanon.cc
.
10. Adding Predefined Named Parameters
The interpreter comes with a set of predefined named parameters for accessing internal state from the NGC language level. These parameters are read-only and global, and hence cannot be assigned to.
Additional parameters may be added by defining a function in the namedparams
module. The name of the function defines the name of the new predefined named parameter, which now can be referenced in arbitrary expressions.
To add or redefine a named parameter:
-
Add a
namedparams
module so it can be found by the interpreter, -
define new parameters by functions (see below). These functions receive
self
(the interpreter instance) as parameter and so can access arbitrary state. Arbitrary Python capabilities can be used to return a value. -
Import that module from the
TOPLEVEL
script.
# namedparams.py # trivial example def _pi(self): return 3.1415926535
Functions in namedparams.py
are expected to return a float or int value. If a string is returned, this sets the interpreter error message and aborts execution.
Ã’nly functions with a leading underscore are added as parameters, since this is the RS274NGC convention for globals.
It is possible to redefine an existing predefined parameter by adding a Python function of the same name to the namedparams
module. In this case, a warning is generated during startup.
While the above example isn’t terribly useful, note that pretty much all of the interpreter internal state is accessible from Python, so arbitrary predicates may be defined this way. For a slightly more advanced example, see tests/remap/predefined-named-params
.
11. Standard Glue routines
Since many remapping tasks are very similar, I’ve started collecting working prolog and epilog routines in a single Python module. These can currently be found in ncfiles/remap_lib/python-stdglue/stdglue.py and provide the following routines:
11.1. T: prepare_prolog
and prepare_epilog
These wrap a NGC procedure for Tx Tool Prepare.
prepare_prolog
The following parameters are made visible to the NGC procedure:
-
#<tool>
- the parameter of theT
word -
#<pocket>
- the corresponding pocket
If tool number zero is requested (meaning Tool unload), the corresponding pocket is passed as -1.
It is an error if:
-
No tool number is given as T parameter,
-
the tool cannot be found in the tool table.
Note that unless you set the [EMCIO] RANDOM_TOOLCHANGER=1
parameter, tool and pocket number are identical, and the pocket number from the tool table is ignored. This is currently a restriction.
prepare_epilog
-
The NGC procedure is expected to return a positive value, otherwise an error message containing the return value is given and the interpreter aborts.
-
In case the NGC procedure executed the T command (which then refers to the built in T behavior), no further action is taken. This can be used for instance to minimally adjust the built in behavior be preceding or following it with some other statements.
-
Otherwise, the
#<tool>
and#<pocket>
parameters are extracted from the subroutine’s parameter space. This means that the NGC procedure could change these values, and the epilog takes the changed values in account. -
Then, the Canon command
SELECT_TOOL(#<tool>)
is executed.
11.2. M6: change_prolog
and change_epilog
These wrap a NGC procedure for M6 Tool Change.
change_prolog
-
If there was no preceding T command which caused a pocket to be selected, the prolog aborts with an error message.
-
If cutter radius compensation is on, the prolog aborts with an error message.
Then, the following parameters are exported to the NGC procedure:
-
#<tool_in_spindle>
: the tool number of the currently loaded tool -
#<selected_tool>
: the tool number selected -
#<selected_pocket>
: the selected tool’s tooldata index
-
The NGC procedure is expected to return a positive value, otherwise an error message containing the return value is given and the interpreter aborts.
-
In case the NGC procedure executed the M6 command (which then refers to the built in M6 behavior), no further action is taken. This can be used for instance to minimally adjust the built in behavior be preceding or following it with some other statements.
-
Otherwise, the
#<selected_pocket>
parameter is extracted from the subroutine’s parameter space, and used to set the interpreter’scurrent_pocket
variable. Again, the procedure could change this value, and the epilog takes the changed value in account. -
Then, the Canon command
CHANGE_TOOL(#<selected_pocket>)
is executed. -
The new tool parameters (offsets, diameter etc) are set.
11.3. G-code Cycles: cycle_prolog
and cycle_epilog
These wrap a NGC procedure so it can act as a cycle, meaning the motion code is retained after finishing execution. If the next line just contains parameter words (e.g. new X,Y values), the code is executed again with the new parameter words merged into the set of the parameters given in the first invocation.
These routines are designed to work in conjunction with an argspec=<words>
parameter. While this is easy to use, in a realistic scenario you would avoid argspec and do a more thorough investigation of the block manually in order to give better error messages.
The suggested argspec is as follows:
REMAP=G<somecode> argspec=xyzabcuvwqplr prolog=cycle_prolog ngc=<ngc procedure> epilog=cycle_epilog modalgroup=1
This will permit cycle_prolog
to determine the compatibility of any axis words give in the block, see below.
cycle_prolog
-
Determine whether the words passed in from the current block fulfill the conditions outlined under Canned Cycle Errors.
-
Export the axis words as
<x>
,#<y>
etc; fail if axis words from different groups (XYZ) (UVW) are used together, or any of (ABC) is given. -
Export L- as
#<l>
; default to 1 if not given. -
Export P- as
#<p>
; fail if p less than 0. -
Export R- as
#<r>
; fail if r not given, or less equal 0 if given. -
Fail if feed rate is zero, or inverse time feed or cutter compensation is on.
-
-
Determine whether this is the first invocation of a cycle G-code, if so:
-
Add the words passed in (as per argspec) into a set of sticky parameters, which is retained across several invocations.
-
-
If not (a continuation line with new parameters) then
-
merge the words passed in into the existing set of sticky parameters.
-
-
Export the set of sticky parameters to the NGC procedure.
cycle_epilog
-
Determine if the current code was in fact a cycle, if so, then
-
retain the current motion mode so a continuation line without a motion code will execute the same motion code.
-
11.4. S (Set Speed) : setspeed_prolog
and setspeed_epilog
TBD
11.5. F (Set Feed) : setfeed_prolog
and setfeed_epilog
TBD
11.6. M61 Set tool number : settool_prolog
and settool_epilog
TBD
12. Remapped code execution
12.1. NGC procedure call environment during remaps
Normally, an O-word procedure is called with positional parameters. This scheme is very limiting in particular in the presence of optional parameters. Therefore, the calling convention has been extended to use something remotely similar to the Python keyword arguments model.
See LINKTO G-code/main Subroutines: sub, endsub, return, call.
12.2. Nested remapped codes
Remapped codes may be nested just like procedure calls - that is, a remapped code whose NGC procedure refers to some other remapped code will execute properly.
The maximum nesting level remaps is currently 10.
12.3. Sequence number during remaps
Sequence numbers are propagated and restored like with O-word calls. See tests/remap/nested-remaps/word
for the regression test, which shows sequence number tracking during nested remaps three levels deep.
12.4. Debugging flags
The following flags are relevant for remapping and Python - related execution:
|
|
traces execution of O-word subroutines |
|
|
traces execution of remap-related code |
|
|
calls to the Python plug in |
|
|
trace named parameter access |
|
|
user-defined - not interpreted by LinuxCNC |
|
|
user-defined - not interpreted by LinuxCNC |
or these flags into the [EMC]DEBUG
variable as needed. For a current list of debug flags see src/emc/nml_intf/debugflags.h.
12.5. Debugging Embedded Python code
Debugging of embedded Python code is harder than debugging normal Python scripts, and only a limited supply of debuggers exists. A working open-source based solution is to use the Eclipse IDE, and the PydDev Eclipse plug in and its remote debugging feature.
To use this approach:
-
Install Eclipse via the the Ubuntu Software Center (choose first selection).
-
Install the PyDev plug in from the Pydev Update Site.
-
Setup the LinuxCNC source tree as an Eclipse project.
-
Start the Pydev Debug Server in Eclipse.
-
Make sure the embedded Python code can find the
pydevd.py
module which comes with that plug in - it is buried somewhere deep under the Eclipse install directory. Set the thepydevd
variable inutil.py
to reflect this directory location. -
Add
import pydevd
to your Python module - see exampleutil.py
andremap.py
. -
Call
pydevd.settrace()
in your module at some point to connect to the Eclipse Python debug server - here you can set breakpoints in your code, inspect variables, step etc. as usual.
pydevd.settrace() will block execution if Eclipse and the Pydev debug server have not been started. |
To cover the last two steps: the o<pydevd>
procedure helps to get into the debugger from MDI mode. See also the call_pydevd
function in util.py
and its usage in remap.involute
to set a breakpoint.
Here’s a screen-shot of Eclipse/PyDevd debugging the involute
procedure from above:
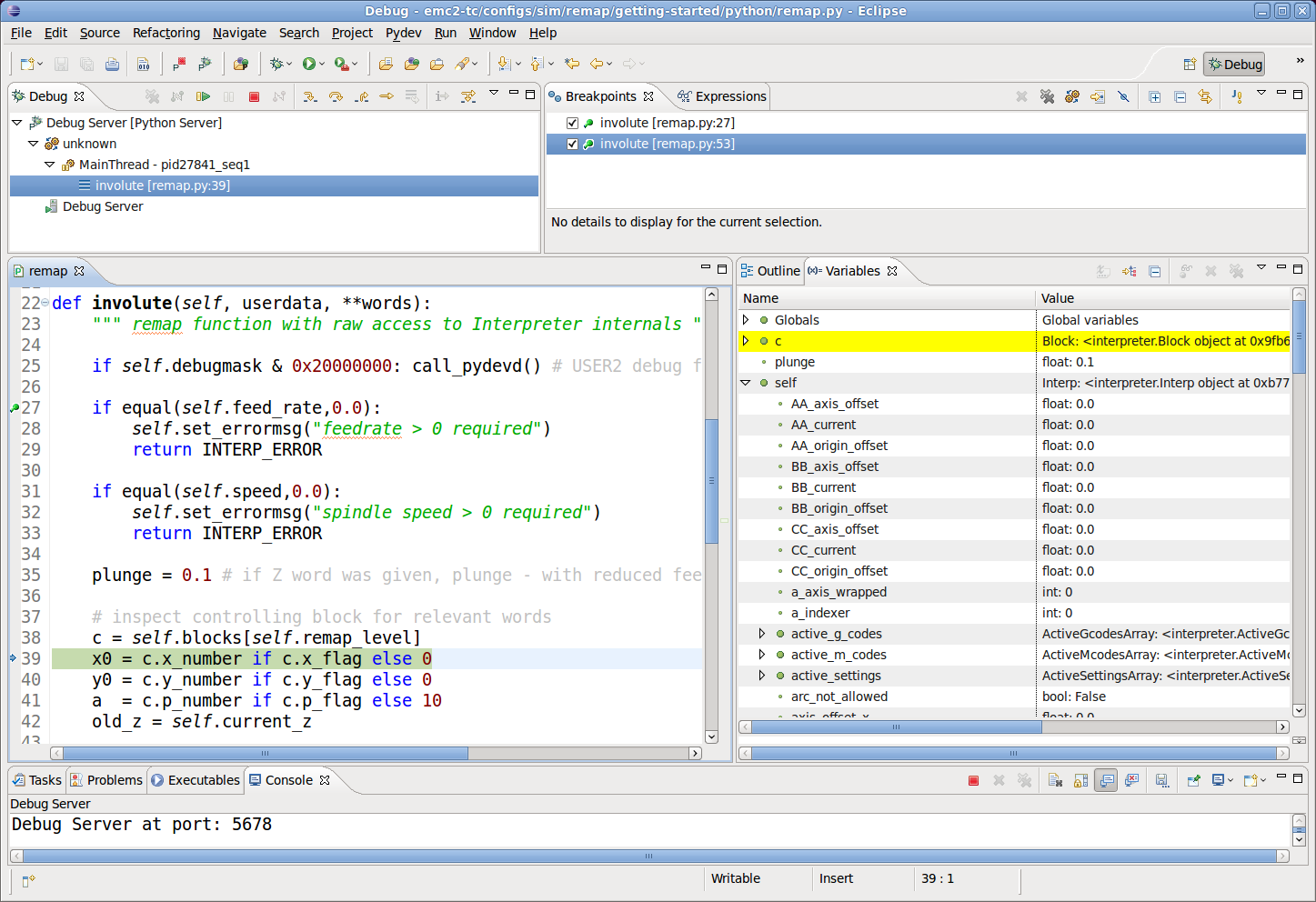
See the Python code in configs/sim/axis/remap/getting-started/python
for details.
13. Axis Preview and Remapped code execution
For complete preview of a remapped code’s tool path some precautions need to be taken. To understand what is going on, let’s review the preview and execution process (this covers the AXIS case, but others are similar):
First, note that there are two independent interpreter instances involved:
-
One instance in the milltask program, which executes a program when you hit the Start button, and actually makes the machine move.
-
A second instance in the user interface whose primary purpose is to generate the tool path preview. This one executes a program once it is loaded, but it doesn’t actually cause machine movements.
Now assume that your remap procedure contains a G38 probe operation, for example as part of a tool change with automatic tool length touch off. If the probe fails, that would clearly be an error, so you’d display a message and abort the program.
Now, what about preview of this procedure? At preview time, of course it is not known whether the probe succeeds or fails - but you would likely want to see what the maximum depth of the probe is, and assume it succeeds and continues execution to preview further movements. Also, there is no point in displaying a probe failed message and aborting during preview.
The way to address this issue is to test in your procedure whether it executes in preview or execution mode. This can be checked for by testing the #<_task>
predefined named parameter - it will be 1 during actual execution and 0 during preview. See configs/sim/axis/remap/manual-toolchange-with-tool-length-switch/nc_subroutines/manual_change.ngc for a complete usage example.
Within Embedded Python, the task
instance can be checked for by testing self.task - this will be 1 in the milltask instance, and 0 in the preview instance(s).
14. Remappable Codes
14.1. Existing codes which can be remapped
The current set of existing codes open to redefinition is:
-
Tx (Prepare)
-
M6 (Change tool)
-
M61 (Set tool number)
-
M0 (pause a running program temporarily)
-
M1 (pause a running program temporarily if the optional stop switch is on)
-
M7 (activate coolant mist)
-
M8 (activate coolant flood)
-
M9 (deactivate coolant mist and flood)
-
M60 (exchange pallet shuttles and then pause a running program temporarily)
-
M62 .. M65 (digital output control)
-
M66 (wait on input)
-
M67, M68 (analog output control)
-
S (set spindle speed)
-
F (set feed)
14.2. Currently unallocated G-codes:
Currently unallocated G-codes (for remapping) must be selected from the blank areas of the following tables. All the listed G-codes are already defined in the current implementation of LinuxCNC and may not be used to remap new G-codes. (Developers who add new G-codes to LinuxCNC are encouraged to also add their new G-codes to these tables.)
# | Gxx | Gxx.1 | Gxx.2 | Gxx.3 | Gxx.4 | Gxx.5 | Gxx.6 | Gxx.7 | Gxx.8 | Gxx.9 |
---|---|---|---|---|---|---|---|---|---|---|
00 |
|
|||||||||
01 |
|
|||||||||
02 |
|
|||||||||
03 |
|
|||||||||
04 |
|
|||||||||
05 |
|
|
|
|
||||||
06 |
||||||||||
07 |
|
|||||||||
08 |
|
|||||||||
09 |
# | Gxx | Gxx.1 | Gxx.2 | Gxx.3 | Gxx.4 | Gxx.5 | Gxx.6 | Gxx.7 | Gxx.8 | Gxx.9 |
---|---|---|---|---|---|---|---|---|---|---|
10 |
|
|||||||||
11 |
||||||||||
12 |
||||||||||
13 |
||||||||||
14 |
||||||||||
15 |
||||||||||
16 |
||||||||||
17 |
|
|
||||||||
18 |
|
|
||||||||
19 |
|
|
# | Gxx | Gxx.1 | Gxx.2 | Gxx.3 | Gxx.4 | Gxx.5 | Gxx.6 | Gxx.7 | Gxx.8 | Gxx.9 |
---|---|---|---|---|---|---|---|---|---|---|
20 |
|
|||||||||
21 |
|
|||||||||
22 |
||||||||||
23 |
||||||||||
24 |
||||||||||
25 |
||||||||||
26 |
||||||||||
27 |
||||||||||
28 |
|
|
||||||||
29 |
# | Gxx | Gxx.1 | Gxx.2 | Gxx.3 | Gxx.4 | Gxx.5 | Gxx.6 | Gxx.7 | Gxx.8 | Gxx.9 |
---|---|---|---|---|---|---|---|---|---|---|
30 |
|
|
||||||||
31 |
||||||||||
32 |
||||||||||
33 |
|
|
||||||||
34 |
||||||||||
35 |
||||||||||
36 |
||||||||||
37 |
||||||||||
38 |
||||||||||
39 |
# | Gxx | Gxx.1 | Gxx.2 | Gxx.3 | Gxx.4 | Gxx.5 | Gxx.6 | Gxx.7 | Gxx.8 | Gxx.9 |
---|---|---|---|---|---|---|---|---|---|---|
40 |
|
|||||||||
41 |
|
|
||||||||
42 |
|
|
||||||||
43 |
|
|
||||||||
44 |
||||||||||
45 |
||||||||||
46 |
||||||||||
47 |
||||||||||
48 |
||||||||||
49 |
|
# | Gxx | Gxx.1 | Gxx.2 | Gxx.3 | Gxx.4 | Gxx.5 | Gxx.6 | Gxx.7 | Gxx.8 | Gxx.9 |
---|---|---|---|---|---|---|---|---|---|---|
50 |
||||||||||
51 |
||||||||||
52 |
||||||||||
53 |
|
|||||||||
54 |
|
|||||||||
55 |
|
|||||||||
56 |
|
|||||||||
57 |
|
|||||||||
58 |
|
|||||||||
59 |
|
|
|
|
# | Gxx | Gxx.1 | Gxx.2 | Gxx.3 | Gxx.4 | Gxx.5 | Gxx.6 | Gxx.7 | Gxx.8 | Gxx.9 |
---|---|---|---|---|---|---|---|---|---|---|
60 |
|
|||||||||
61 |
|
|
||||||||
62 |
||||||||||
63 |
||||||||||
64 |
|
|||||||||
65 |
||||||||||
66 |
||||||||||
67 |
||||||||||
68 |
||||||||||
69 |
# | Gxx | Gxx.1 | Gxx.2 | Gxx.3 | Gxx.4 | Gxx.5 | Gxx.6 | Gxx.7 | Gxx.8 | Gxx.9 |
---|---|---|---|---|---|---|---|---|---|---|
70 |
|
|||||||||
71 |
|
|
|
|||||||
72 |
|
|
|
|||||||
73 |
||||||||||
74 |
||||||||||
75 |
||||||||||
76 |
|
|||||||||
77 |
||||||||||
78 |
||||||||||
79 |
# | Gxx | Gxx.1 | Gxx.2 | Gxx.3 | Gxx.4 | Gxx.5 | Gxx.6 | Gxx.7 | Gxx.8 | Gxx.9 |
---|---|---|---|---|---|---|---|---|---|---|
80 |
|
|||||||||
81 |
|
|||||||||
82 |
|
|||||||||
83 |
|
|||||||||
84 |
|
|||||||||
85 |
|
|||||||||
86 |
|
|||||||||
87 |
|
|||||||||
88 |
|
|||||||||
89 |
|
# | Gxx | Gxx.1 | Gxx.2 | Gxx.3 | Gxx.4 | Gxx.5 | Gxx.6 | Gxx.7 | Gxx.8 | Gxx.9 |
---|---|---|---|---|---|---|---|---|---|---|
90 |
|
|
||||||||
91 |
|
|
||||||||
92 |
|
|
|
|
||||||
93 |
|
|||||||||
94 |
|
|||||||||
95 |
|
|||||||||
96 |
|
|||||||||
97 |
|
|||||||||
98 |
|
|||||||||
99 |
|
14.3. Currently unallocated M-codes:
These M-codes are currently undefined in the current implementation of LinuxCNC and may be used to define new M-codes. (Developers who define new M-codes in LinuxCNC are encouraged to remove them from this table.)
# | Mx0 | Mx1 | Mx2 | Mx3 | Mx4 | Mx5 | Mx6 | Mx7 | Mx8 | Mx9 |
---|---|---|---|---|---|---|---|---|---|---|
00-09 |
||||||||||
10-19 |
|
|
|
|
|
|
|
|
|
|
20-29 |
|
|
|
|
|
|
|
|
|
|
30-39 |
|
|
|
|
|
|
|
|
|
|
40-49 |
|
|
|
|
|
|
|
|
||
50-59 |
|
|
|
|
|
|
||||
60-69 |
||||||||||
70-79 |
|
|
|
|
|
|
||||
80-89 |
|
|
|
|
|
|
|
|
|
|
90-99 |
|
|
|
|
|
|
|
|
|
|
All M-codes from M100
to M199
are user-defined M-codes already, and should not be remapped.
All M-codes from M200
to M999
are available for remapping.
15. A short survey of LinuxCNC program execution
To understand remapping of codes it might be helpful to survey the execution of task
and interpreter as far as it relates to remapping.
15.1. Interpreter state
Conceptually, the interpreter’s state consist of variables which fall into the following categories:
-
Configuration information (typically from INI file)
-
The 'World model' - a representation of actual machine state
-
Modal state and settings - refers to state which is carried over between executing individual NGC codes - for instance, once the spindle is turned on and the speed is set, it remains at this setting until turned off. The same goes for many codes, like feed, units, motion modes (feed or rapid) and so forth.
-
Interpreter execution state - Holds information about the block currently executed, whether we are in a subroutine, interpreter variables, etc. . Most of this state is aggregated in a - fairly unsystematic -
structure _setup
(see interp_internals.hh).
15.2. Task and Interpreter interaction, Queuing and Read-Ahead
The task
part of LinuxCNC is responsible for coordinating actual machine commands - movement, HAL interactions and so forth. It does not by itself handle the RS274NGC language. To do so, task
calls upon the interpreter to parse and execute the next command - either from MDI or the current file.
The interpreter execution generates canonical machine operations, which actually move something. These are, however, not immediately executed but put on a queue. The actual execution of these codes happens in the task
part of LinuxCNC: canon commands are pulled off that interpreter queue, and executed resulting in actual machine movements.
This means that typically the interpreter is far ahead of the actual execution of commands - the parsing of the program might well be finished before any noticeable movement starts. This behavior is called read-ahead.
15.3. Predicting the machine position
To compute canonical machine operations in advance during read ahead, the interpreter must be able to predict the machine position after each line of G-code, and that is not always possible.
Let’s look at a simple example program which does relative moves (G91), and assume the machine starts at x=0,y=0,z=0. Relative moves imply that the outcome of the next move relies on the position of the previous one:
Here the interpreter can clearly predict machine positions for each line:
After N20: x=10 y=-5 z=20; after N30: x=10 y=15 z=15; after N40: x=10 y=15 z=45 and so can parse the whole program and generate canonical operations well in advance.
15.4. Queue-busters break position prediction
However, complete read ahead is only possible when the interpreter can predict the position impact for every line in the program in advance. Let’s look at a modified example:
To pre-compute the move in N90, the interpreter would need to know where the machine is after line N80 - and that depends on whether the probe command succeeded or not, which is not known until it is actually executed.
So, some operations are incompatible with further read-ahead. These are called queue busters, and they are:
-
Reading a HAL pin’s value with M66: value of HAL pin not predictable.
-
Loading a new tool with M6: tool geometry not predictable.
-
Executing a probe with G38.n: final position and success/failure not predictable.
15.5. How queue-busters are dealt with
Whenever the interpreter encounters a queue-buster, it needs to stop read ahead and wait until the relevant result is available. The way this works is:
-
When such a code is encountered, the interpreter returns a special return code to
task
(INTERP_EXECUTE_FINISH). -
This return code signals to
task
to stop read ahead for now, execute all queued canonical commands built up so far (including the last one, which is the queue buster), and then synchronize the interpreter state with the world model. Technically, this means updating internal variables to reflect HAL pin values, reload tool geometries after an M6, and convey results of a probe. -
The interpreter’s synch() method is called by
task
and does just that - read all the world model actual values which are relevant for further execution. -
At this point,
task
goes ahead and calls the interpreter for more read ahead - until either the program ends or another queue-buster is encountered.
15.6. Word order and execution order
One or several words may be present on an NGC block if they are compatible (some are mutually exclusive and must be on different lines). The execution model however prescribes a strict ordering of execution of codes, regardless of their appearance on the source line (G-code Order of Execution).
15.7. Parsing
Once a line is read (in either MDI mode, or from the current NGC file), it is parsed and flags and parameters are set in a struct block (struct _setup, member block1). This struct holds all information about the current source line, but independent of different ordering of codes on the current line: As long as several codes are compatible, any source ordering will result in the same variables set in the struct block. Right after parsing, all codes on a block are checked for compatibility.
15.8. Execution
After successful parsing the block is executed by execute_block(), and here the different items are handled according to execution order.
If a "queue buster" is found, a corresponding flag is set in the interpreter state (toolchange_flag, input_flag, probe_flag) and the interpreter returns an INTERP_EXECUTE_FINISH return value, signaling stop readahead for now, and resynch to the caller (task
). If no queue busters are found after all items are executed, INTERP_OK is returned, signalling that read-ahead may continue.
When read ahead continues after the synch, task
starts executing interpreter read() operations again. During the next read operation, the above mentioned flags are checked and corresponding variables are set (because the a synch() was just executed, the values are now current). This means that the next command already executes in the properly set variable context.
15.9. Procedure execution
O-word procedures complicate handling of queue busters a bit. A queue buster might be found somewhere in a nested procedure, resulting in a semi-finished procedure call when INTERP_EXECUTE_FINISH is returned. Task makes sure to synchronize the world model, and continue parsing and execution as long as there is still a procedure executing (call_level > 0).
15.10. How tool change currently works
The actions happening in LinuxCNC are a bit involved, but it is necessary to get the overall idea what currently happens, before you set out to adapt those workings to your own needs.
Note that remapping an existing code completely disables all internal processing for that code. That means that beyond your desired behavior (probably described through an NGC O-word or Python procedure), you need to replicate those internal actions of the interpreter, which together result in a complete replacement of the existing code. The prolog and epilog code is the place to do this.
Several processes are interested in tool information: task
and its interpreter, as well as the user interface. Also, the halui process.
Tool information is held in the emcStatus structure, which is shared by all parties. One of its fields is the toolTable array, which holds the description as loaded from the tool table file (tool number, diameter, frontangle, backangle and orientation for lathe, tool offset information).
The authoritative source and only process actually setting tool information in this structure is the iocontrol process. All others processes just consult this structure. The interpreter holds actually a local copy of the tool table.
For the curious, the current emcStatus structure can be accessed by Python statements. The interpreter’s perception of the tool currently loaded for instance is accessed by:
;py,from interpreter import *
;py,print(this.tool_table[0])
You need to have LinuxCNC started from a terminal window to see the results.
15.11. How T
x (Prepare Tool) works
T
x commandAll the interpreter does is evaluate the toolnumber parameter, looks up its corresponding tooldata index, remembers it in the selected_pocket
variable for later, and queues a canon command (SELECT_TOOL). See Interp::convert_tool_select in src/emc/rs274/interp_execute.cc.
When task
gets around to handle a SELECT_TOOL, it sends a EMC_TOOL_PREPARE message to the iocontrol
process, which handles most tool-related actions in LinuxCNC.
In the current implementation, task
actually waits for iocontrol
to complete the changer positioning operation, which is not necessary IMO since it defeats the idea that changer preparation and code execution can proceed in parallel.
When iocontrol
sees the select pocket command, it does the related HAL pin wiggling - it sets the "tool-prep-number" pin to indicate which tool is next, raises the "tool-prepare" pin, and waits for the "tool-prepared" pin to go high.
When the changer responds by asserting "tool-prepared", it considers the prepare phase to be completed and signals task
to continue. Again, this wait is not strictly necessary IMO.
Tx
See the Python functions prepare_prolog
and prepare_epilog
in nc_files/remap_lib/python-stdglue/stdglue.py
.
15.12. How M6 (Change tool) works
You need to understand this fully before you can adapt it. It is very relevant to writing a prolog and epilog handler for a remapped M6. Remapping an existing codes means you disable the internal steps taken normally, and replicate them as far as needed for your own purposes.
Even if you are not familiar with C, I suggest you look at the Interp::convert_tool_change code in src/emc/rs274/interp_convert.cc.
When the interpreter sees an M6, it:
-
checks whether a
T
command has already been executed (test settings->selected_pocket to be >= 0) and fail with Need tool prepared -Txx
- for toolchange message if not. -
check for cutter compensation being active, and fail with Cannot change tools with cutter radius compensation on if so.
-
stop the spindle except if the "TOOL_CHANGE_WITH_SPINDLE_ON" INI option is set.
-
generate a rapid Z up move if if the "TOOL_CHANGE_QUILL_UP" INI option is set.
-
if TOOL_CHANGE_AT_G30 was set:
-
move the A, B and C indexers if applicable
-
generate rapid move to the G30 position
-
-
execute a CHANGE_TOOL canon command, with the selected pocket as a parameter. CHANGE_TOOL will:
-
generate a rapid move to TOOL_CHANGE_POSITION if so set in INI
-
enqueue an EMC_TOOL_LOAD NML message to
task
.
-
-
set the numberer parameters 5400-5413 according to the new tool
-
signal to
task
to stop calling the interpreter for readahead by returning INTERP_EXECUTE_FINISH since M6 is a queue buster.
task
does when it sees a CHANGE_TOOL commandAgain, not much more than passing the buck to iocontrol
by sending it an EMC_TOOL_LOAD message, and waiting until iocontrol
has done its thing.
-
it asserts the "tool-change" pin
-
it waits for the "tool-changed" pin to become active
-
when that has happened:
-
deassert "tool-change"
-
set "tool-prep-number" and "tool-prep-pocket" pins to zero
-
execute the load_tool() function with the pocket as parameter.
-
The last step actually sets the tooltable entries in the emcStatus structure. The actual action taken depends on whether the RANDOM_TOOLCHANGER INI option was set, but at the end of the process toolTable[0] reflects the tool currently in the spindle.
When that has happened:
-
iocontrol
signalstask
to go ahead. -
task
tells the interpreter to execute a synch() operation, to see what has changed. -
The interpreter synch() pulls all information from the world model needed, among it the changed tool table.
From there on, the interpreter has complete knowledge of the world model and continues with read ahead.
M6
See the Python functions change_prolog
and change_epilog
in nc_files/remap_lib/python-stdglue/stdglue.py
.
15.13. How M61
(Change tool number) works
M61
requires a non-negative `Q`parameter (tool number). If zero, this means unload tool, else set current tool number to Q.
M61
An example Python redefinition for M61
can be found in the set_tool_number
function in nc_files/remap_lib/python-stdglue/stdglue.py
.
16. Status
-
The RELOAD_ON_CHANGE feature is fairly broken. Restart after changing a Python file.
17. Changes
-
The method to return error messages and fail used to be self.set_errormsg(text) followed by return INTERP_ERROR. This has been replaced by merely returning a string from a Python handler or oword subroutine. This sets the error message and aborts the program. Previously there was no clean way to abort a Python O-word subroutine.
18. Debugging
In the [EMC] section of the INI file the DEBUG parameter can be changed to get various levels of debug messages when LinuxCNC is started from a terminal.
Debug level, 0 means no messages. See src/emc/nml_intf/debugflags.h for others
DEBUG = 0x00000002 # configuration
DEBUG = 0x7FFFDEFF # no interp,oword
DEBUG = 0x00008000 # py only
DEBUG = 0x0000E000 # py + remap + Oword
DEBUG = 0x0000C002 # py + remap + config
DEBUG = 0x0000C100 # py + remap + Interpreter
DEBUG = 0x0000C140 # py + remap + Interpreter + NML msgs
DEBUG = 0x0000C040 # py + remap + NML
DEBUG = 0x0003E100 # py + remap + Interpreter + oword + signals + namedparams
DEBUG = 0x10000000 # EMC_DEBUG_USER1 - trace statements
DEBUG = 0x20000000 # EMC_DEBUG_USER2 - trap into Python debugger
DEBUG = 0x10008000 # USER1, PYTHON
DEBUG = 0x30008000 # USER1,USER2, PYTHON # USER2 will cause involute to try to connect to pydev
DEBUG = 0x7FFFFFFF # All debug messages